I have been developing WordPress plugin for a while now and it seems like there are always some correct and better ways of writing a particular code in WordPress than mindlessly trying to substitute it with pure PHP. However, these WordPress codes can only be found through countless reading and analyzing of codes from other WordPress sources. In this article, i will present as many tips and tricks i have seen in WordPress that can be very useful for wordpress plugin development.
Find Plugin Directory and URL With WordPress
Previously, i used to hard code the directory by using PHP function. However, after realize there is a better alternative in WordPress, i changed the way i find the plugin directory and URL.
In PHP,
$url = get_bloginfo('url')."/wp-content/plugins/plugin-name/images/hello.jpg";
$directory = dirname(__FILE__)."/plugin-name/images/hello.jpg";
Note: Using dirname(__FILE__) might not always end up on the plugin folder.
In WordPress,
$url = WP_PLUGIN_URL."/plugin-name/images/hello.jpg";
$directory = WP_PLUGIN_DIR."/plugin-name/images/hello.jpg";
Import CSS or JavaScript in WordPress
We love to code these import statement out to the function that performed the action. It can be on the admin page, write post page, home page, etc. But the correct way is to use WordPress action hook and built-in method.
Import CSS/JavaScript to Admin page
function hpt_loadcss()
{
wp_enqueue_style('hpt_ini', WP_PLUGIN_URL.'/hungred-post-thumbnail/css/hpt_ini.css');
}
function hpt_loadjs()
{
wp_enqueue_script('jquery');
wp_enqueue_script('hpt_ini', WP_PLUGIN_URL.'/hungred-post-thumbnail/js/hpt_ini.js');
}
add_action('admin_print_scripts', 'hpt_loadjs');
add_action('admin_print_styles', 'hpt_loadcss');
Import to theme page
function ham_add_style()
{
$style = WP_PLUGIN_URL . '/hungred-ads-manager/css/ham_template.css';
$location = WP_PLUGIN_DIR . '/hungred-ads-manager/css/ham_template.css';
if ( file_exists($location) ) {
wp_register_style('template', $style);
wp_enqueue_style( 'template');
}
}
add_action('wp_print_styles', 'ham_add_style');
Both ways utilize the wp_enqueue_style/wp_enqeue_script method and action hook to import stylesheet and JavaScript properly into WordPress.
Separate Plugin Admin Code
This is only necessary if you are building a large plugin for WordPress. It is efficient to separate the admin codes from others by placing it on an external file so that the admin codes will not be complied by PHP when non-admin user or visitors are accessing your website.
if (is_admin())
include(‘admin.php’);
Secure your WordPress Query
Security something important for all of us. WordPress has a function escape() in their global variable $wpdb. It is best to use this for all data query in your WordPress to better secure your SQL query with the database to prevent any form of security attack. below shows an example,
$welcome_name = "Mr. WordPress";
$welcome_text = "Congratulations, you just completed the installation!";
$insert = "INSERT INTO " . $table_name .
" (time, name, text) " .
"VALUES ('" . time() . "','" . $wpdb->escape($welcome_name) . "','" . $wpdb->escape($welcome_text) . "')";
$results = $wpdb->query( $insert );
You may want to visit the presentation slide that have some interesting WordPress function used for securitySecure Coding with WordPress – WordCamp SF 2008 Slides
Use WordPress For Table Prefix
Never hard code your table prefix in WordPress! WordPress provides a variable in its global variable $wpdb that allows you to easily retrieve your table prefix.
global $wpdb;
$table_name = $wpdb->prefix . "liveshoutbox";
Get Absolute Path In WordPress
In WordPress, you can get the absolute path through the constant ABSPATH which is defined in WordPress.
require_once( ABSPATH . '/wp-includes/classes.php' );
require_once( ABSPATH . '/wp-includes/functions.php' );
require_once( ABSPATH . '/wp-includes/plugin.php' );
Determine Whether a Table Exist In WordPress
Wonder how to determine whether a table exist in your WordPress? You can use the following method to detect whether a particular table exist.
global $wpdb;
$table_name = $wpdb->prefix . "mytable";
if($wpdb->get_var("show tables like '$table_name'") == $table_name) {
echo 'table exist!';
}
Always Record Table Version
This is an important tips. Always remember to record the version of your plugin table, so you can use that information later if you need to update the table structure. This can help in upgrading your table structure of the plugin in the future.
add_option("hungred_db_version", "1.0");
Create Table Using WordPress Method
This is important for many WordPress developers out there. Although we can create a table using the following method,
$table = $wpdb->prefix."ham_form";
$structure = "CREATE TABLE `".$table."` (
ham_id DOUBLE NOT NULL DEFAULT 1,
ham_textarea longtext NOT NULL,
ham_display longtext NOT NULL,
UNIQUE KEY id (ham_id)
);";
$wpdb->query($structure);
Great! A table is created! Now, tell me how are you going to change this structure in the future? A better alternative is to use the function dbDelta in WordPress.
$table = $wpdb->prefix."ham_form";
$structure = "CREATE TABLE `".$table."` (
ham_id DOUBLE NOT NULL DEFAULT 1,
ham_textarea longtext NOT NULL,
ham_display longtext NOT NULL,
UNIQUE KEY id (ham_id)
);";
require_once(ABSPATH . 'wp-admin/includes/upgrade.php');
dbDelta($structure);
Directly from WordPress,
The dbDelta function examines the current table structure, compares it to the desired table structure, and either adds or modifies the table as necessary, so it can be very handy for updates (see wp-admin/upgrade-schema.php for more examples of how to use dbDelta). Note that the dbDelta function is rather picky, however. For instance:
* You have to put each field on its own line in your SQL statement.
* You have to have two spaces between the words PRIMARY KEY and the definition of your primary key.
* You must use the key word KEY rather than its synonym INDEX
Hence, any update on the structure of the table will result in a change on the user plugin as well.
Use Nonces During Form Submission
Nonces are used as a security related protection to prevent attacks and mistakes. You can use Nonces to enhance your WordPress form. here is an example,
<form ...>
<?php
if ( function_exists('wp_nonce_field') )
wp_nonce_field('hungred-post-form'+$uniqueobj);
?>
</form>
We are just using the method wp_nonce_field in WordPress to create a nonce field on the above example. Next, we will need to validate whether the nonce is valid by using the following method after the user have submitted the form. This should be placed before any action began.
<?php check_admin_referer('hungred-post-form'+$uniqueobj); ?>
Pretty easy for enhancing form in your WordPress plugin. But this is not all you can do. There is also Link nonce protection where link is attached with a Nonces. You can read more about Nonces from the below link.
They have better explanation and example to understand Nonce.
Speed up your WordPress plugin development with Ubiquity Firefox add-on
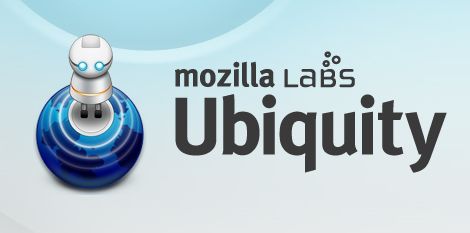
Ubiquity is a Mozilla Firefox add-on, developed by Mozilla Labs. It allows you to search WordPress and PHP (PHP) documentation in an instant. Safe time on Google, more time on development 😀